创建 npm 工具库
打包工具的选择
如果我们需要构建一个简单的小型应用并让它快速运行起来,可以使用
Parcel
;如果需要构建一个类库只需要导入很少第三方库,可以使用
Rollup
;如果需要构建一个复杂的应用,需要集成很多第三方库,并且需要代码分拆、HMR等功能,推荐使用
Webpack
[3]。
所以,在开发工具库时,我们选择 rollup
作为打包工具。
初始化
1 | mkdir wow-tool |
安装 rollup
将 rollup 安装为本地开发依赖
1 | # npm |
安装 rollup 插件
先安装如下rollup插件:
插件包名 | 作用 |
---|---|
@rollup/plugin-node-resolve | 使用 nodejs的解析算法,使得可以在 node_modules 中使用第三方包 |
@rollup/plugin-commonjs | 将 CommonJS 模块转成 ES 模块 |
@rollup/plugin-alias | 在打包的时候创建别名 |
@rollup/plugin-replace | 在打包时替换目标字符串 |
@rollup/plugin-eslint | 代码规范化 |
@rollup/plugin-babel | 与 babel 无缝集成,将 ES6 代码转换成 ES5 |
rollup-plugin-terser | 通过使用 terser 引擎,缩减打包后的大小 |
rollup-plugin-clear | 在编译前清空输出目录 |
@rollup/plugin-json | 将 json 文件转成 ES 模块 |
rollup-plugin-serve | 创建开发服务 |
rollup-plugin-livereload | 实时重载代码修改 |
rollup-plugin-filesize | 在终端中显示包文件的大小 |
安装命令如下:
1 | # npm |
插件的具体作用参考:rollup官方插件库
配置 rollup
- 根据开发环境区分不同的配置
- 设置对应的
npm script
- 输出不同规范的产物:umd、umd.min、cjs、esm
- 兼容
jest
不支持es module
的问题
1 | mkdir config |
rollup.config.base.js
1 | import { nodeResolve } from '@rollup/plugin-node-resolve' // 解析 node_modules 中的模块 |
修改包名:
1 | // 此处 pkgName 要修改成自己的包名 |
rollup.config.dev.js
1 | import baseConfig from './rollup.config.base' |
rollup.config.prod.js
1 | import baseConfig from './rollup.config.base' |
配置 prettier
prettier 主要用于代码格式校验和修正。
1 | # --save-exact 添加准确的版本号,例如:"webpack": "^5.1.3",添加--save-exact后将没有 ^ 号 |
配置 eslint
eslint 主要用于代码质量的校验。
安装
1 | // 在开发环境中安装 esLint |
安装插件并配置
1 | // 使用 standard 规范 |
如果有更复杂的需求,可以安装 eslint-config-prettier 来禁用 eslint 与 prettier 之间冲突的配置。
配置 babel
1 | npm i -D @babel/core @babel/preset-env babel-plugin-transform-async-to-promises |
单元测试
test 目录下创建 xxx.test.js(xxx 和 源码中的文件名保持一致)
- 选用
jest
做单元测试 - 配置
eslint
的jest
环境 - 解决
jest
不支持es module
的问题
1 | npm i -D jest |
更新忽略文件
.gitignore
1 | # `n 代表换行 |
.npmignore
1 | # 新建文件 |
README.md
添加徽标
- GitHub徽标官网是shields.io
- 普通徽标
1 | https://img.shields.io/badge/{徽标标题}-{徽标内容}-{徽标颜色}.svg |
- 动态徽标
1 | https://img.shields.io/github/issues/{github用户名}/{仓库名}.svg |
git 提交校验
安装下列包:
包名 | 作用 |
---|---|
husky | 关联git的hook与项目,可以实现在提交时校验提交信息的规范性 |
@commitlint/config-conventional | 代码提交 message 规范校验格式库 |
@commitlint/cli | 代码提交 message 规范校验 |
commitizen | 代码交互提交 |
cz-conventional |
完整安装及配置:
1 | npm install --save-dev husky @commitlint/config-conventional @commitlint/cli commitizen cz-conventional-changelog |
常用的commitlint type类别:
- build:发布
- chore:构建过程或辅助工具的变动
- ci:合并其它贡献者的代码变化(continuous integration)
- docs:文档(documentation)
- feat:新功能(feature)
- fix:修补bug
- perf:
- refactor:重构(即不是新增功能,也不是修改bug的代码变动)
- style: 格式(不影响代码运行的变动)
- test:增加测试
例:
git commit -m 'feat: 增加 xxx 功能' git commit -m 'bug: 修复 xxx 功能'
配置package.json
经过上述配置,我们还需要配置一些 npm 脚本来运行,如下:
1 | "scripts": { |
完整版:
1 | { |
编写功能
上述配置完成后,就可以开始编写功能了。
入口函数为 src/index.js
,该入口在
rollup.config.base.js
中定义的。
1 | // src/index.js |
编写测试
针对上述的 humanStorageSize
方法编写一个单元测试,单元编写参考:rollup-jest 、jest
使用示例:
1 | import path from 'path' |
humanStorageSize 测试示例:
1 | // 新建文件 |
执行测试:
1 | // 运行测试 |
测试结果:
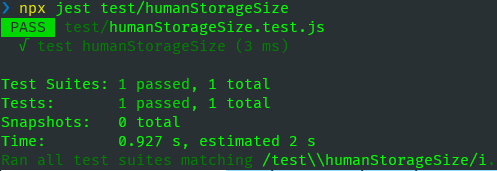
通过 Git 管理包
如果公司没有私有包服务器,同时也不想将包发到 npm 上,可以发布到私有 Git 仓库里,然后直接从 Git 安装包。>> 用 git 管理私有包
发布到 npm
发布到 verdaccio
参考
本文主要参考以下文章,在此致以诚挚谢意!